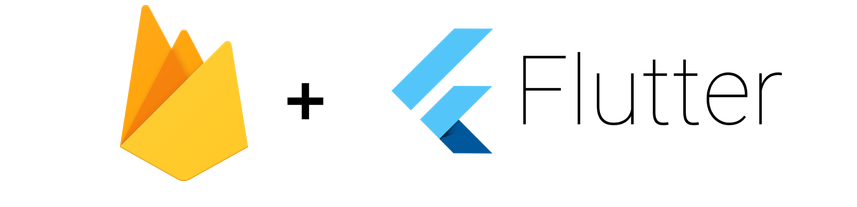
Step 1: Create a Firebase Project
- Go to the Firebase Console and click on "Add project."
- Follow the prompts to set up your project. Once done, click on "Continue" and then "Create project."
Step 2: Add your Flutter App to Firebase
- After creating the project, click on "Add app" and select the Flutter icon.
- Register your app by providing a nickname and package name (make sure it matches your Flutter project).
- Download the
google-services.json
file and place it in theandroid/app
directory. - For iOS, click on "Register App" and follow the prompts. Download the
GoogleService-Info.plist
file and add it to theios/Runner
directory of your Flutter project.
Step 3: Configure Firebase in your Flutter Project
Open your pubspec.yaml file and add the Firebase dependencies:
dependencies: firebase_core: ^latest_version firebase_auth: ^latest_version cloud_firestore: ^latest_version
- Run flutter pub get in your terminal to install the new dependencies.
Step 4: Initialize Firebase in your Flutter App
Import the Firebase packages in your main.dart:
import 'package:firebase_core/firebase_core.dart';
Initialize Firebase in the main function:
void main() async { WidgetsFlutterBinding.ensureInitialized(); await Firebase.initializeApp(); runApp(MyApp()); }
Step 5: Use Firebase Services in your Flutter App
Authentication
Import the necessary packages:
import 'package:firebase_auth/firebase_auth.dart';
Implement authentication:
final FirebaseAuth _auth = FirebaseAuth.instance; Future<void> signInWithGoogle() async { try { // Implement Google Sign-In logic here UserCredential userCredential = await _auth.signInWithGoogle(); print("Signed in: ${userCredential.user.displayName}"); } catch (e) { print("Error during Google Sign-In: $e"); } }
Firestore Database
Import the necessary packages:
import 'package:cloud_firestore/cloud_firestore.dart';
Use Firestore in your code:
final FirebaseFirestore _firestore = FirebaseFirestore.instance; Future<void> addUserToFirestore(String username, String email) { return _firestore.collection('users').add({ 'username': username, 'email': email, }); }
Step 6: Test your Firebase Integration
Run your Flutter app, and ensure that Firebase is initialized without any errors. Test the authentication and Firestore features to confirm their functionality.
Congratulations! You've successfully integrated Firebase into your Flutter project. This sets the foundation for incorporating various Firebase services into your app to enhance its functionality.